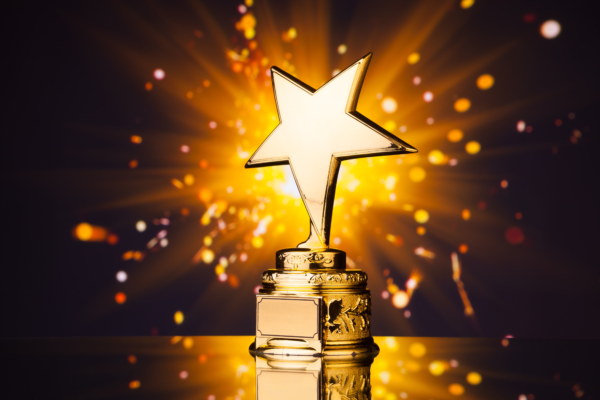
Astro’s variable scope
When you need front-end interactivity, you can set it up like we’ve done using a Reactive framework or with vanilla JavaScript. Either way, Astro works on the server side. A good way to drive this point home is to look at Astro’s support for variables that can be inserted into the HTML templates. For example, we could create a starting value in index.astro
like so:
---
import ReactCounter from '../components/ReactCounter.jsx';
import SvelteCounter from '../components/SvelteCounter.svelte';
const startingValue = Math.floor(Math.random() * 100);
---
// …
<h3>This is on the server: {startingValue}</h3>
This displays the random value generated on the server on the client page. Remember, though, that it’s all done on the server. If we wanted to use this value on the client, we could pass it into the components as a parameter. For example, we could make the Svelte component accept a parameter like so:
<script>
export let startingValue;
let count = startingValue;
function handleClick() {
count += 1;
}
</script>
<div>
<p>Count: {count}</p>
<button on:click={handleClick}>Increment</button>
</div>
Notice the export let startingValue
syntax, which is Svelte-speak for exposing a pass-in parameter to its parent. To use this back on the index page you’d type: