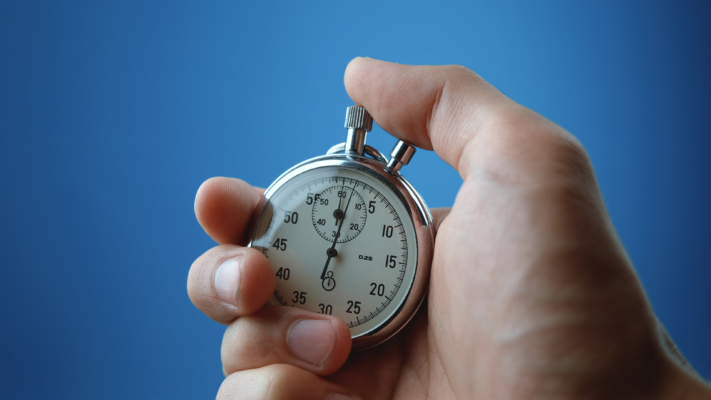
Now, if you’re thinking of using asyncio.sleep()
in a loop to wait constantly for some external condition … don’t. While you can do this, in theory, it’s a clumsy way to handle that situation. Passing an asyncio.Event
object to a task is a better approach, allowing you to just wait for the Event
object to change.
Async and file I/O
Network I/O in async can be made not to block, as described above. But local file I/O blocks the current thread by default. One workaround is to delegate the file I/O operation to another thread using asyncio.to_thread()
, so that other tasks in the event loop can still be processed.
Another way to handle file I/O in async is with the third-party aiofiles
library. This gives you high-level async constructs for opening, reading, and writing files—e.g., async with aiofiles.open("myfile.txt") as f:
. If you don’t mind having it as a dependency in your project, it’s an elegant way to deal with this issue.